Introduction to Short Strangle Strategy
Anticipating stability but caught off guard by market swings? The Short Strangle strategy is built for range-bound conditions, allowing traders to sell out-of-the-money (OTM) call and put options. This approach provides a wider breakeven range while collecting premium. In this blog, we’ll break down how it works, risk management techniques, and ways to adapt it to different market conditions.
Understanding the Short Strangle
A Short Strangle involves selling both a call and a put at out-of-the-money (OTM) strike prices with the same expiration date.
It can be used in two ways:
-
Long Strangle: Buying both options to profit from high volatility and significant price movement.
-
Short Strangle: Selling both options to generate premium income in a low-volatility environment.
The current strategy builds on the traditional short strangle by integrating risk controls to optimize returns while managing downside risk effectively.
How the Short Strangle Strategy Works
1. Entry Criteria
Eligible Markets: This strategy is ideal for liquid indices like NSE’s NIFTY 50, NIFTY Bank, and US-listed options like QQQ, AAPL, and GOOGL which offer low bid-ask spreads for efficient trade execution.
Strike Price Selection: Short Strangles involve selling OTM Call and Put options positioned around the ATM, with adjustable width based on risk preference. Narrower strikes collect higher premiums but limit the profitable range, while wider strikes lower premiums but extend the breakeven zone.
Expiry Selection:
- Weekly Expiry: Captures rapid theta decay and allows for frequent trades.
- Monthly Expiry: Slower theta decay, offering stability and fewer adjustments (Theta decay and its impact on strategy duration is discussed later).
Lot Size & Positioning: The number of contracts (lots) traded is set within the strategy parameters, ensuring a structured approach to risk exposure.
Execution Parameters: The strategy is adaptable to various timeframes, but a 1-minute candle interval is recommended for precise monitoring and timely execution in response to market conditions.
2. Exit Rules & Re-Entry Strategy
The strategy exits when:
- Profit Target Reached: Exits when the net premium declines below a set percentage, capturing gains.
- Stop-Loss Triggered: Automatically exits when net premium surpasses a defined threshold to prevent significant losses.
Re-entry Conditions
Once an exit condition is met, the strategy re-enters using the same entry logic, ensuring continued participation in range-bound markets. Traders can also apply rolling adjustments to shift the short strikes further OTM if the market is trending unexpectedly.
Alternative Exit Strategies
- Partial Profit Booking: If one leg moves favorably, traders can book profits on that leg while keeping the other open.
- Time-Based Exit: Instead of a strict stop-loss, traders may exit after a certain percentage of the expiry period has passed.
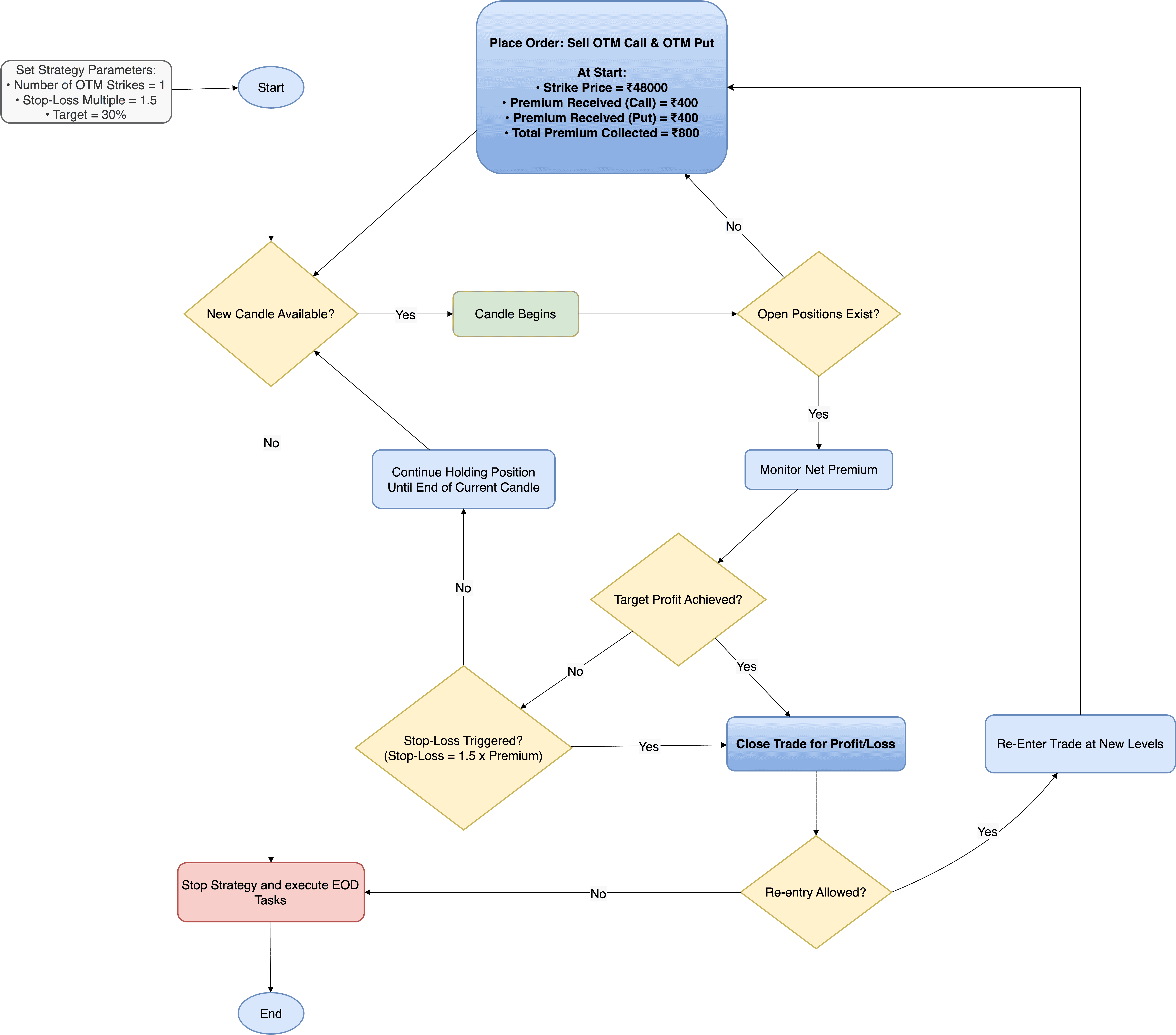
Figure 1: Short Strangle Strategy Execution Flowchart
(Values align with the first sample in the upcoming Profit & Loss Calculations section)
This section covers the Python implementation of a Short Strangle with defined strike distance and automated risk control.
Selecting Instruments and Strikes for Entry
def strategy_select_instruments_for_entry(self, candle, instruments_bucket):
selected_instruments, meta = [], []
for instrument in instruments_bucket:
self.logger.debug(
f"Checking entry conditions for base instrument: {instrument} | "
f"Determining OTM option instruments and verifying if CE/PE orders are already placed."
)
leg_wise_list = [
("CE", self.child_instrument_main_order_ce.get(instrument)),
("PE", self.child_instrument_main_order_pe.get(instrument))
]
for tradingsymbol_suffix, main_order in leg_wise_list:
if main_order:
continue # Skip processing if an order has already been placed for this leg
self.options_instruments_set_up_all_expiries(instrument, tradingsymbol_suffix, self.broker.get_ltp(instrument)) # Set up option instruments for available expiries
child_instrument = self.get_child_instrument_details(instrument, tradingsymbol_suffix, OptionsStrikeDirection.OTM.value, self.number_of_strikes) # Retrieve OTM child instrument details for the given instrument
# Map the base instrument to its corresponding child instrument in the instruments' mapper. This allows tracking of relationships between base and child instruments for further processing.
self.instruments_mapper.add_mappings(instrument, child_instrument)
selected_instruments.append(child_instrument)
meta.append({"action": ActionConstants.ENTRY_SELL, "base_instrument": instrument, "tradingsymbol_suffix": tradingsymbol_suffix})
return selected_instruments, meta
Code Explanation:
1. Setting Up Available Expiries:
self.options_instruments_set_up_all_expiries(instrument, tradingsymbol_suffix, self.broker.get_ltp(instrument))
-
This function fetches the latest available option expiry dates and selects the most liquid expiry contract, which is by default the earliest monthly expiry as discussed before.
-
Uses real-time LTP (Last Traded Price) as an input, ensures that decisions are based on the most recent market price and not on stale data.
2. OTM Strike Selection Logic
The system dynamically selects OTM options based on a predefined strike distance from ATM:
child_instrument = self.get_child_instrument_details(
instrument, tradingsymbol_suffix, OptionsStrikeDirection.OTM.value, self.number_of_strikes)
- The self.number_of_strikes parameter controls trade-off between premium collection (lower strikes) and breakeven width (higher strikes).
3. Instrument Mapping for Order Tracking
self.instruments_mapper.add_mappings(instrument, child_instrument)
- This is crucial for tracking base instruments and their option legs, ensuring that the system can later match which option belongs to which underlying security.
Creating an Order for a Position
def strategy_enter_position(self, candle, instrument, meta):
child_instrument = instrument
_order = self.broker.SellOrderRegular(instrument=child_instrument, order_code=self.order_code, order_variety=BrokerOrderVarietyConstants.MARKET, quantity=self.number_of_lots * child_instrument.lot_size)
if self.check_order_placed_successfully(_order):
(self.child_instrument_main_order_ce if meta["tradingsymbol_suffix"] == "CE" else self.child_instrument_main_order_pe)[meta["base_instrument"]] = _order
else:
# Protection logic incase any of the legs fail to get placed - this will help avoid having naked positions
self.logger.critical('Order placement failed for one of the legs. Exiting position for other leg, if possible and stopping strategy.')
base_instrument = self.instruments_mapper.get_base_instrument(child_instrument)
self.exit_all_positions_for_base_instrument(base_instrument)
raise ABSystemExit
return _order
Code Explanation:
1. Using Market Orders for Execution
_order = self.broker.SellOrderRegular(
instrument=child_instrument,
order_code=self.order_code,
order_variety=BrokerOrderVarietyConstants.MARKET,
quantity=self.number_of_lots * child_instrument.lot_size)
-
Market Order ensures immediate execution at the best available price.
-
self.number_of_lots x child_instrument.lot_size guarantees that the correct lot size is executed, preventing mismatched order sizing.
2. Ensuring One-Sided Position Risk is Avoided
if not self.check_order_placed_successfully(_order):
self.exit_all_positions_for_base_instrument(base_instrument)
raise ABSystemExit
- If an order fails to execute, the other leg (CE or PE) is forcefully exited to prevent unintentional directional exposure.
Exit Strategy: Understanding Stop-Loss and Profit Targets
def check_exit_condition(self, main_order_ce, main_order_pe):
""" Determines if the strategy should exit based on stoploss or target. Returns True if exit condition is met. """
ltp_ce = self.broker.get_ltp(main_order_ce.instrument)
ltp_pe = self.broker.get_ltp(main_order_pe.instrument)
total_ltp = ltp_ce + ltp_pe
net_premium_cost = main_order_ce.entry_price + main_order_pe.entry_price
stop_loss_premium = net_premium_cost * self.stoploss_multiplier
target_premium = net_premium_cost * (1 - self.target_percentage / 100)
self.logger.debug(f"LTP (CE) : {ltp_ce}, LTP (PE) : {ltp_pe}, "
f"Net Entry Premium : {net_premium_cost} | Current Net Premium : {total_ltp} "
f"Stop-loss Threshold : {stop_loss_premium} | Target Threshold : {target_premium}")
target_profit_condition = total_ltp < target_premium
if target_profit_condition:
self.logger.debug(f"Target profit reached: Current Net Premium ({total_ltp}) dropped below Target Threshold ({target_premium}). Exiting position.")
return True
stop_loss_condition = total_ltp > stop_loss_premium
if stop_loss_condition:
self.logger.debug(f"Stop-loss triggered: Current Net Premium ({total_ltp}) exceeded Stop-loss Threshold ({stop_loss_premium}). Exiting position.")
return True
Code Explanation:
1. Dynamic Calculation of Stop-Loss and Profit Targets
stop_loss_premium = net_premium_cost * self.stoploss_multiplier
target_premium = net_premium_cost * (1 - self.target_percentage / 100)
-
Stop-loss premium is calculated as a multiplication factor of the initial net premium (net_premium_cost x stoploss_multiplier).
-
Target premium is derived as a percentage-based reduction of the entry premium.
Why Is This Critical in a Short Strangle?
- Without target/risk control, the trade may stay open too long, increasing overnight exposure. A structured exit mechanism reduces this risk.
2. Exit Conditions – Locking Profits or Cutting Losses
target_profit_condition = total_ltp < target_premium
if target_profit_condition:
return True
stop_loss_condition = total_ltp > stop_loss_premium
if stop_loss_condition:
return True
The conditions target_profit_condition and stop_loss_condition evaluates premium fluctuations in real time and triggers exits accordingly.
Validation in Short Strangles
def validate_parameters(self):
""" Validates required strategy parameters. """
check_argument(self.strategy_parameters, "extern_function", lambda x: len(x) >= 3, err_message="Need 3 parameters for this strategy: \n(1) NUMBER_OF_STRIKES \n(2) STOPLOSS_MULTIPLIER \n(3) TARGET_PERCENTAGE")
# Validate number of strikes
check_argument(self.number_of_strikes, "extern_function", is_positive_int, "Value should be positive integer" )
# Validate expiry dates
if len(self.get_allowed_expiry_dates()) != self.number_of_allowed_expiry_dates:
self.logger.info(f"Allowed expiry dates: {self.number_of_allowed_expiry_dates}, got {len(self.get_allowed_expiry_dates())}. Exiting...")
raise SystemExit
# Validate parameters
for param in (self.target_percentage, self.stoploss_multiplier):
check_argument(param, "extern_function", is_nonnegative_int_or_float, "Value should be >0.0")
Code Explanation:
1. Ensuring Required Parameters are Present
Why It Matters:
For a Short Strangle strategy to function correctly, it requires three key parameters:
-
NUMBER_OF_STRIKES: Determines how far the options are from the ATM price.
-
STOPLOSS_MULTIPLIER: Defines the risk limit.
-
TARGET_PERCENTAGE: Specifies the profit-taking threshold.
If any of these parameters are missing, the strategy cannot execute as intended.
Validation Control:
check_argument(self.strategy_parameters, "extern_function", lambda x: len(x) >= 3,
err_message="Need 3 parameters for this strategy: \n(1) NUMBER_OF_STRIKES \n(2) STOPLOSS_MULTIPLIER \n(3) TARGET_PERCENTAGE")
2. Preventing Imbalanced Strike Widths
Why It Matters:
In a Short Strangle, traders must correctly define two different strike prices:
- Out-of-the-Money (OTM) Call (higher strike).
- Out-of-the-Money (OTM) Put (lower strike).
A missing or incorrectly set strike width could result in a Short Straddle instead of a Strangle, increasing risk.
Validation Control:
check_argument(self.number_of_strikes, "extern_function", is_positive_int, "Value should be positive integer")
- Ensures NUMBER_OF_STRIKES is a positive integer, preventing execution errors or unintended strategy misfires.
3. Handling Expiry Mismatches to Prevent Empty Positions
Why It Matters:
Short Strangles rely on short-term expiries to benefit from theta decay. However, if a broker’s API fails to return expiry dates, the system may attempt to execute trades that do not exist, causing capital stagnation.
Validation Control:
if len(self.get_allowed_expiry_dates()) != self.number_of_allowed_expiry_dates:
self.logger.info(f"Allowed expiry dates: {self.number_of_allowed_expiry_dates}, got {len(self.get_allowed_expiry_dates())}. Exiting...")
raise SystemExit
-
This also ensures trades are not placed on non-trading days.
-
Automatically exits if expiry dates are missing, preventing capital lock-up.
4. Preventing Illogical Stop-Loss and Target Settings
Why It Matters:
In a Short Strangle:
- Profit is limited to the premium received, but risk is theoretically unlimited.
- Setting an unrealistic stop-loss or profit target could cause erratic trade behavior.
Risk Example:
If a trader accidentally sets:
self.strategy_parameters = {
"STOPLOSS_MULTIPLIER": -2,
"TARGET_PERCENTAGE": 50
}
Validation Control:
for param in (self.target_percentage, self.stoploss_multiplier):
check_argument(param, "extern_function", is_nonnegative_int_or_float, "Value should be >0.0")
- Ensures STOPLOSS_MULTIPLIER and TARGET_PERCENTAGE are positive and realistic, preventing execution errors.
💡 Want to see the complete strategy? Check Out The Full Implementation Here!
Straddle vs. Strangle: Which Strategy is Right for You?
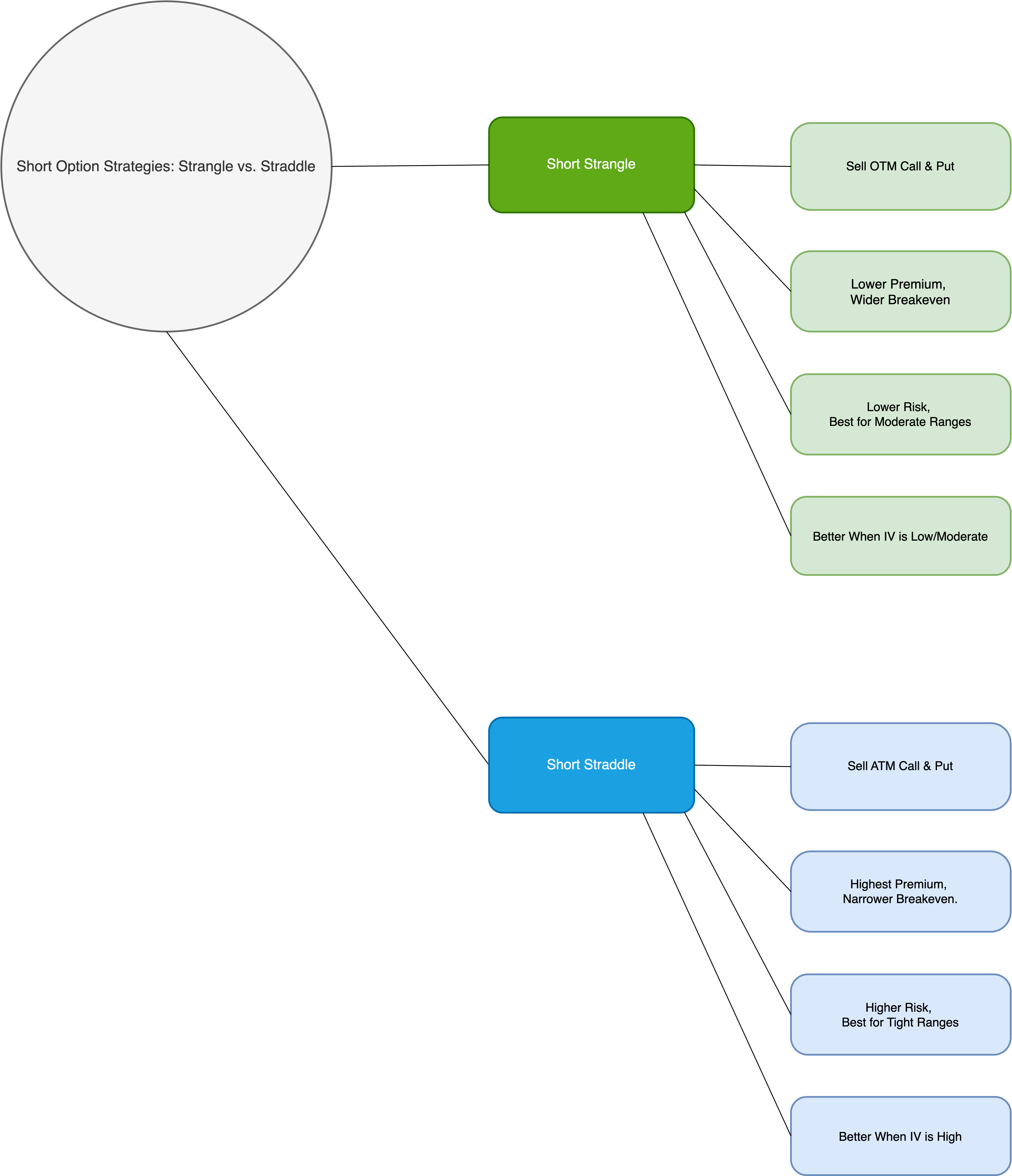
Figure 2 : Short Strangle vs. Short Straddle Mind Tree
The mind tree diagram above provides a structured decision-making roadmap to help traders choose between a Short Strangle and a Short Straddle. Both strategies are designed for range-bound markets, but they differ in terms of risk exposure, premium collected, and breakeven levels. Choosing the right strategy depends on market conditions, implied volatility, and margin requirements.
When to Choose a Short Strangle
✅ Use a Short Strangle when:
-
The market is range-bound but not too tight, allowing for moderate price fluctuations.
-
IV is moderate, making options expensive, allowing for good premium collection while selling farther OTM.
-
You prefer less risk than a Straddle, with a wider breakeven range to absorb small market moves.
-
The underlying asset has historically stable trends and is less prone to sudden spikes or collapses.
-
You want a theta-positive strategy (one that benefits from steady time decay, generating income as option premiums decrease) with lower gamma risk (reduced sensitivity to sudden price movements due to selling non-ATM options, making it less volatile) than a Straddle.
❌ Avoid a Short Strangle if:
-
IV is too low, as it won’t generate enough premium to justify the trade.
-
The asset is highly volatile and prone to sudden breakouts beyond OTM strikes.
-
You need maximum premium collection (a Straddle would be better).
When to Choose a Short Straddle
✅ Use a Short Straddle when:
-
You expect the underlying asset to stay in a very tight range near the ATM strike.
-
IV is low, meaning options are underpriced, making a Short Straddle more attractive than a Strangle.
-
You want to maximize premium collection, even if it means higher gamma risk and narrower breakevens.
-
The asset has historically low volatility, with minimal directional bias over the short term.
-
You can handle the higher margin requirements that come with ATM option selling.
❌ Avoid a Short Straddle if:
-
The asset has a history of strong trends or breakouts.
-
IV is high, which increases the risk of a large move against your position.
-
You prefer a wider breakeven range with less risk (a Strangle may be better).
Time Decay (Theta) Behavior
The following graph illustrates how Short Strangles experience more gradual time decay (theta) compared to Short Straddles, making them more stable over time.
Figure 3: Theta Decay: Short Strangle vs. Short Straddle
-
Short Strangle: More gradual decay, allowing for a smoother premium collection over time.
-
Short Straddle: Faster time decay due to ATM positioning, but higher risk if price moves.
Final Takeaway
-
Choose a Short Strangle if IV is high, you prefer wider breakevens, and expect moderate movement.
-
Choose a Short Straddle if IV is low, you want maximum premium, and expect minimal price movement.
-
Strangles offer more stability due to slower theta decay, whereas Straddles decay faster but carry higher risk.
Other Market Conditions Where Strangles Work Best
While Short Strangles are ideal for range-bound markets, they also work well in specific scenarios, including post-earnings consolidations and mid-term trading.
- Post-Earnings Plays: Stocks or indices tend to consolidate after earnings releases, making Strangles a great play.
- Mid-Term Trading (Not Just Intraday): Strangles can be used for longer timeframes since their wider strike selection makes them more forgiving compared to Straddles.
Profit & Loss Calculations
Medium-Risk Short Strangle Strategy
Key Parameters:
-
Target Profit (target_percentage): 30% of the total premium received
-
Stop-Loss (stop_loss_multiplier): 1.5 x the total premium received, capping the maximum loss.
-
Instrument: NIFTY Bank Index
-
ATM Options Expiry Type: Nearest Monthly Expiry
-
Strikes (number_of_strikes): 1 strike away from ATM (OTM Call & Put)
Calculations:
-
Total Premium Received:
Premium from Call + Premium from Put = ₹400 + ₹400 = ₹800
-
Target Profit:
800 × 0.3 = ₹240
So, we exit when the profit reaches + ₹240.
-
Stop-Loss (1.5 x Total Premium Received):
800 × 1.5 = ₹1200
-
Max Loss Capped at: -₹400 (since ₹800 was already received in premiums)
P&L Outcomes:
Underlying Spot Price | P&L Without Stop-Loss | Adjusted P&L (With Stop-Loss) |
---|---|---|
₹47040 (Price based on Target) | +₹240 (Target Profit) | +₹240 |
₹47200 or ₹48800 (Breakeven) | 0 | 0 |
₹46800 or ₹49200 (Stop-Loss Triggered) | Unlimited | -₹400 (Capped) |
If the price stays between ₹47200 and ₹48800, the trade remains profitable. Beyond ₹46800 or below ₹49200, the stop-loss limits losses to -₹400 (₹800 - ₹1200).
High-Risk Short Strangle Strategy
Key Parameters:
-
Target Profit (target_percentage): 40% of the total premium received
-
Stop-Loss (stop_loss_multiplier): 1.5 x the total premium received, capping the maximum loss.
-
Instrument: NIFTY Bank Index
-
ATM Options Expiry Type: Nearest Monthly Expiry
-
Strikes (number_of_strikes): 2 strikes away from ATM (OTM Call & Put)
Calculations:
-
Total Premium Received:
Premium from Call + Premium from Put = ₹200 + ₹200 = ₹400
-
Target Profit:
400 × 0.4 = ₹160
So, we exit when the profit reaches + ₹160.
-
Stop-Loss (1.5 x Total Premium Received):
400 × 1.5 = ₹600
-
Max Loss Capped at: - ₹200 (since ₹400 was already received in premiums)
P&L Outcomes:
Underlying Spot Price | P&L Without Stop-Loss | Adjusted P&L (With Stop-Loss) |
---|---|---|
₹47760 (Price based on Target) | +₹160 (Target Profit) | +₹160 |
₹47600 or ₹48400 (Breakeven) | 0 | 0 |
₹47400 or ₹48600 (Stop-Loss Triggered) | Unlimited | -₹200 (Capped) |
A Medium-Risk Short Strangle offers smaller but steadier profits, reducing stop-loss hits, while a High-Risk version has higher potential returns but a greater likelihood of reaching stop-loss.
Analysing the Short Strangles Payoff Diagram
The payoff diagram below illustrates the profit and loss dynamics of a Short Strangle strategy, covering both medium-risk and high-risk variations.
Figure 4: Profit and Loss Diagram: Medium vs High Risk Short Strangles
- The green curve represents potential profit, peaking when the underlying asset remains near the ATM strike at expiry.
- The red curve indicates losses, which increase as the price moves further from the strike.
- Stop-loss levels (red horizontal lines) define automatic exit points to prevent excessive drawdowns.
- Target profit levels (dashed green lines) mark early exit triggers when a set profit percentage is reached.
To demonstrate how the Short Strangle strategy applies beyond indices, let’s analyse a trade on AAPL (Apple Inc.), a U.S.-listed stock, with wider strike selection and adjusted risk parameters.
AAPL Stock Short Strangle Strategy
Figure 5: Profit and Loss Diagram: AAPL Short Strangle
Key Parameters:
-
Target Profit (target_percentage): 50% of the total premium received
-
Stop-Loss (stop_loss_multiplier): 2x the total premium received
-
Instrument: AAPL (Apple Inc.)
-
ATM Options Expiry Type: Nearest Monthly Expiry
-
Strikes (number_of_strikes): 5 strikes away from ATM (OTM Call & Put)
Calculations:
-
Premium Collected (Call + Put): $2.50 + $2.50 = $5.00
-
Target Profit: $5.00 × 0.5 = +$2.50 (Exit when reached)
-
Stop-Loss: $5.00 × 2 = $10, loss capped at -$5.00
Underlying Price | P&L Without Stop-Loss | P&L With Stop-Loss |
---|---|---|
$192.5 (Target Profit Price) | +$2.50 | +$2.50 |
$195 or $205 (Breakeven) | $0 | $0 |
$190 or $210 (Stop-Loss) | Unlimited Loss | -$5.00 (Capped) |
Customise the Strategy with Your Own Parameters!
Traders can fine-tune strike selection, expiry choice, stop-loss levels, and profit targets based on risk tolerance.
👉 For testing on the Phoenix platform of AlgoBulls, head over to our site now!
👉 A Jupyter notebook for this strategy is coming soon. Meanwhile, check out All Available Notebooks for Testing Custom Strategies!
Final Thoughts
Beyond standard exit mechanisms, traders can further refine risk control through rolling adjustments and structural modifications like converting to an Iron Condor. These adjustments help manage risk dynamically as market conditions evolve.
Advanced Risk Management
Short Strangles can be adjusted in two ways:
-
Rolling Adjustments:
-
Moves short strikes further OTM near expiry or when price nears a strike, widening the breakeven range while lowering potential income.
-
Reduces stop-loss hits but lowers premium collection.
-
-
Iron Condor Conversion (Risk-Defined Strategy):
-
Adds protective long options to define maximum risk while keeping a wide profit range.
-
Slightly reduces profit potential but provides a defined loss limit.
-
Iron Condor Setup:
- Sell an OTM Call and an OTM Put (Short Strangle setup)
- Buy a further OTM Call (above short call)
- Buy a further OTM Put (below short put)
-
Before vs. After – Converting Short Strangle to Iron Condor
Strategy | Max Loss | Breakeven Range | Premium Collected |
---|---|---|---|
Short Strangle | Unlimited | Wide | High |
Iron Condor (After Adding Protective Legs) | Limited | Slightly Narrower | Lower |
The Short Strangle strategy provides a structured approach for range-bound markets, balancing premium collection with controlled risk. Traders can select a higher-risk (greater exposure, increased premium) or lower-risk (limited losses, reduced premium) approach based on their risk tolerance.