Expecting the market to stay put? The Short Straddle turns stagnation into opportunity - selling both call and put at the same strike to collect premiums while price action stays muted.
This blog breaks down execution, risk controls, and code logic to help you manage risk and generate steady returns in sideways markets.
Understanding the Short Straddle Strangle Strategy
A straddle involves trading both a call and put option at the same strike price and expiration date. It can be used in two ways:
- Long Straddle: Buying both options to profit from high volatility.
- Short Straddle: Selling both options to generate premium income in a low-volatility environment.
This strategy refines the traditional short straddle by integrating risk controls to optimize returns and limit losses.
How the Strategy Works
1. Entry Criteria
-
Eligible Markets: This strategy works best with highly liquid indices and stocks, including NSE’s NIFTY 50, NIFTY Bank, and US options like SPX, NVIDIA, and AAPL, ensuring smooth execution and optimal pricing.
-
Expiry Selection: Weekly expiry for quicker premium decay or Monthly expiry for better liquidity and slightly longer holding periods.
(By default, the later code implementation selects the most recent monthly expiry, but it can be customised to use weekly expiries as well.) -
Strike Price Selection: The system selects ATM (At-The-Money) options for both Calls and Puts based on the current market price of the index.
-
Lot Size & Positioning: The number of contracts (lots) entered is defined in the strategy parameters, ensuring that risk exposure is controlled.
-
Additional Parameters: The strategy can work on any candle. However, it is recommended to use a 1-minute candle interval to enable high-frequency monitoring and quick execution based on market conditions.
Next, let’s explore how the strategy manages exits using predefined stop-loss and target thresholds.
2. Exit Rules
The trade automatically exits when:
- The net premium falls below a predefined target percentage of the entry value, securing profits.
- The net premium exceeds the stop-loss threshold, preventing large drawdowns.
3. Re-entry Conditions
Once an exit is triggered, the strategy resets and re-enters under the same conditions, ensuring continued market participation.
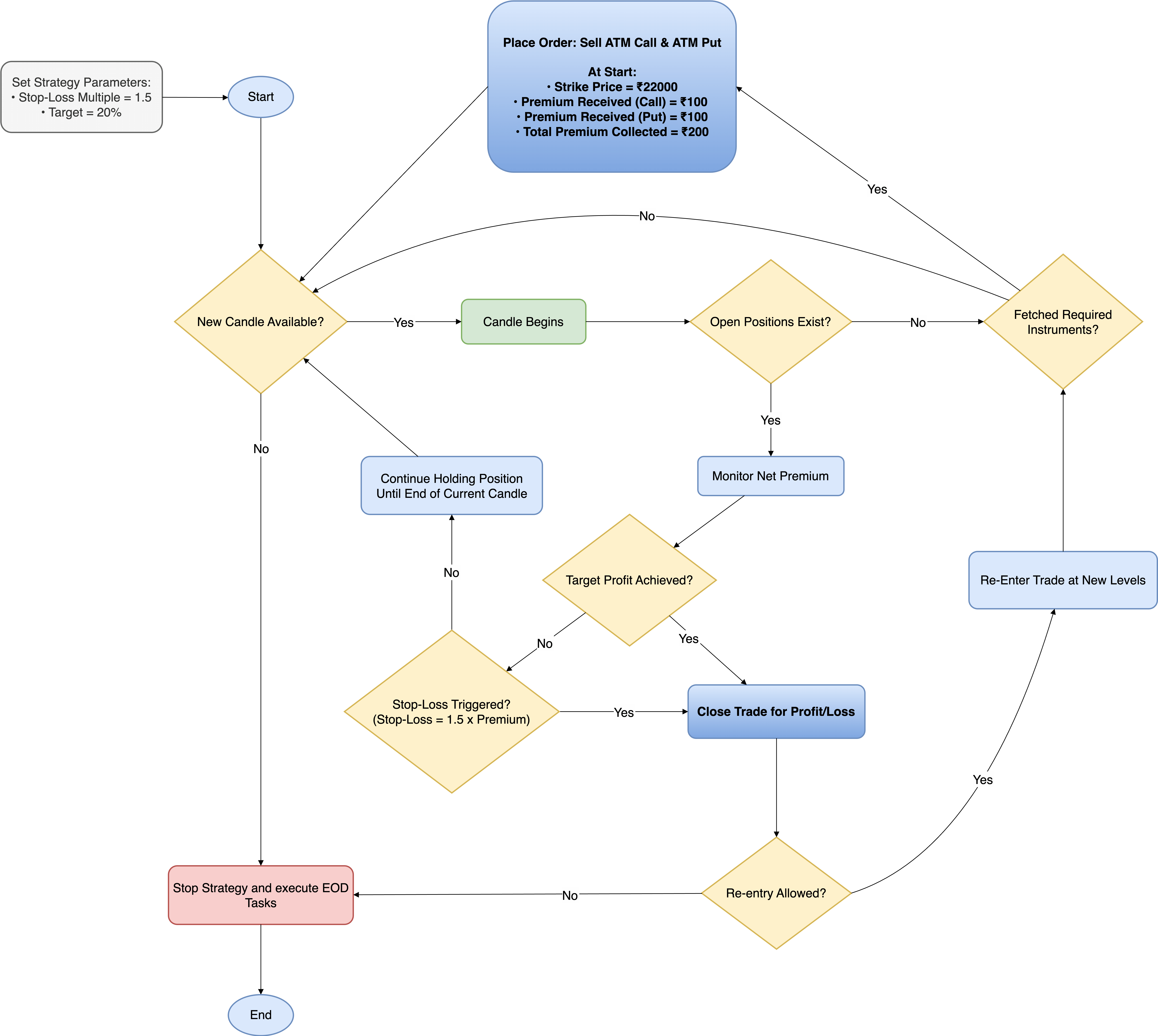
Figure 1: Short Straddle Strategy Flowchart
(Values align with the first sample in the upcoming Profit & Loss Calculations section)
Now that we’ve gone through the strategy mechanics, let’s look at how the core logic is implemented in code:
Selecting Instruments for Entry
def strategy_select_instruments_for_entry(self, candle, instruments_bucket):
selected_instruments, meta = [], []
for instrument in instruments_bucket:
self.logger.debug(
f"Checking entry conditions for base instrument: {instrument} | "
f"Determining ATM option instruments and verifying if CE/PE orders are already placed."
)
leg_wise_list = [
("CE", self.child_instrument_main_order_ce.get(instrument)),
("PE", self.child_instrument_main_order_pe.get(instrument))
]
for tradingsymbol_suffix, main_order in leg_wise_list:
if main_order:
continue # Skip processing if an order has already been placed for this leg
self.options_instruments_set_up_all_expiries(instrument, tradingsymbol_suffix, self.broker.get_ltp(instrument)) # Set up option instruments for available expiries
child_instrument = self.get_child_instrument_details(instrument, tradingsymbol_suffix, OptionsStrikeDirection.ATM.value, 0) # Retrieve ATM child instrument details for the given instrument
# Map the base instrument to its corresponding child instrument in the instruments' mapper. This allows tracking of relationships between base and child instruments for further processing.
self.instruments_mapper.add_mappings(instrument, child_instrument)
selected_instruments.append(child_instrument)
meta.append({"action": ActionConstants.ENTRY_SELL, "base_instrument": instrument, "tradingsymbol_suffix": tradingsymbol_suffix})
return selected_instruments, meta
Code Explanation:
The strategy_select_instruments_for_entry
function identifies ATM Call and Put options for executing a short straddle.
1. Why Check for Existing Orders?
for tradingsymbol_suffix, main_order in leg_wise_list:
if main_order:
continue # Skip if an order has already been placed
- Prevents duplicate trades, ensuring orders are only placed for new legs.
- Ensures the strategy does not reprocess instruments already in position.
2. Why Set Up All Expiries?
self.options_instruments_set_up_all_expiries(
instrument, tradingsymbol_suffix, self.broker.get_ltp(instrument)
)
- Ensures the system checks all available expiries before selecting the correct one.
- Prevents failures if the default expiry is unavailable.
3. What Does ENTRY_SELL Mean?
meta.append({"action": ActionConstants.ENTRY_SELL, "base_instrument": instrument, "tradingsymbol_suffix": tradingsymbol_suffix})
- Indicates shorting the option contract to later buy it back at a lower price, aligning with the intraday short straddle strategy.
Exit Condition Check
def check_exit_condition(self, main_order_ce, main_order_pe):
""" Determines if the strategy should exit based on stoploss or target. Returns True if the exit condition is met. """
ltp_ce = self.broker.get_ltp(main_order_ce.instrument)
ltp_pe = self.broker.get_ltp(main_order_pe.instrument)
total_ltp = ltp_ce + ltp_pe
net_premium_cost = main_order_ce.entry_price + main_order_pe.entry_price
stop_loss_premium = net_premium_cost * self.stoploss_multiplier
target_premium = net_premium_cost * (1 - self.target_percentage / 100)
self.logger.debug(f"LTP (CE) : {ltp_ce}, LTP (PE) : {ltp_pe}, "
f"Net Entry Premium : {net_premium_cost} | Current Net Premium : {total_ltp} "
f"Stop-loss Threshold : {stop_loss_premium} | Target Threshold : {target_premium}")
target_profit_condition = total_ltp < target_premium
if target_profit_condition:
self.logger.debug(f"Target profit reached: Current Net Premium ({total_ltp}) dropped below Target Threshold ({target_premium}). Exiting position.")
return True
stop_loss_condition = total_ltp > stop_loss_premium
if stop_loss_condition:
self.logger.debug(f"Stop-loss triggered: Current Net Premium ({total_ltp}) exceeded Stop-loss Threshold ({stop_loss_premium}). Exiting position.")
return True
Code Explanation:
The check_exit_condition
function decides whether to exit based on stop-loss or target profit.
1. Why Use LTP Instead of Close Price?
ltp_ce = self.broker.get_ltp(main_order_ce.instrument)
ltp_pe = self.broker.get_ltp(main_order_pe.instrument)
total_ltp = ltp_ce + ltp_pe
- LTP enables immediate exits, while the close price causes delays until the next candle.
2. Exit Logic: Why It’s Structured This Way
if total_ltp < target_premium:
return True # Exit (target profit met)
if total_ltp > stop_loss_premium:
return True # Exit (stop-loss triggered)
- Target profit is checked first to lock in gains. The trade stays open if no exit condition is met.
Validating Strategy Parameters in the Short Straddle Strategy
The following code snippet validates key inputs before execution:
def validate_parameters(self):
""" Validates required strategy parameters. """
check_argument(self.strategy_parameters, "extern_function", lambda x: len(x) >= 2, err_message="Need 2 parameters for this strategy: \n(1) STOPLOSS_MULTIPLIER \n(2) TARGET_PERCENTAGE")
# Validate expiry dates
if len(self.get_allowed_expiry_dates()) != self.number_of_allowed_expiry_dates:
self.logger.info(f"Allowed expiry dates: {self.number_of_allowed_expiry_dates}, got {len(self.get_allowed_expiry_dates())}. Exiting...")
raise SystemExit
# Validate parameters
for param in (self.target_percentage, self.stoploss_multiplier):
check_argument(param, "extern_function", is_nonnegative_int_or_float, "Value should be >0.0")
Code Explanation:
The validate_parameters
function ensures that all necessary inputs are correctly set before execution.
Failure Case 1: Missing Required Parameters
self.strategy_parameters = {} # Empty dictionary (parameters missing)
validate_parameters()
- Error: "Need 2 parameters for this strategy: STOPLOSS_MULTIPLIER & TARGET_PERCENTAGE"
- Reason: The function requires at least two parameters, but none were provided.
Failure Case 2: Stop-Loss Multiplier Set to a Negative Value
self.strategy_parameters = {"STOPLOSS_MULTIPLIER": -1, "TARGET_PERCENTAGE": 20}
validate_parameters()
- Error: "Value should be >0.0"
- Reason: A negative stop-loss multiplier is invalid as it would result in illogical trade exits.
Failure Case 3: Expiry Date Mismatch
self.number_of_allowed_expiry_dates = 1
self.get_allowed_expiry_dates = lambda: [] # Returns an empty list (no expiry dates available)
validate_parameters()
- Error: "Allowed expiry dates: 1, got 0. Exiting..."
- Reason: The system expects one expiry date but found none, triggering an exit.
💡 Want to see the complete strategy? Check out the full implementation here.
Interpreting the Payoff Diagram
The following payoff diagram represents the profit and loss dynamics of a short straddle strategy. The values used refer to those used in the flowchart.
Figure 2: Profit and Loss Diagram: Low Risk Version
-
The green curve represents potential profits, peaking at the strike price, where both options expire worthless.
-
The red curve indicates capped losses, flattening at a predefined stop-loss level. However, actual stop-losses may be larger since they are executed one candle later.
- To reduce execution delays, we transition from a candle-based approach to live tick-level price-based execution. More on this in another blog.
-
Dashed lines highlight key levels:
- Breakeven points, where the profit/loss curve crosses zero.
- Target profit level, marking an early exit trigger.
Now, let’s break down profit and loss calculations for different scenarios.
Profit & Loss Calculations
1. Low-Risk Straddle Strategy
The previous diagram sets up an example for the low-risk version of the short straddle.
Key Parameters:
- Target Profit (target_percentage): 20% of the total premium received
- Stop-Loss (stop_loss_multiplier): 1.5 x the total premium received, capping the maximum loss.
- Instrument: NIFTY 50 Index
- ATM Options Expiry Type: Nearest Monthly Expiry
Calculations:
-
Total Premium Received: Premium from Call + Premium from Put = ₹100 + ₹100 = ₹200
-
Target Profit: 200 × 0.2 = ₹40 So, we exit when the profit reaches + ₹40.
-
Stop-Loss (1.5 x Total Premium Received): 200 × 1.5 = ₹300
-
Max Loss Capped at: - ₹100 (since ₹200 was already received in premiums)
P&L Outcomes:
Underlying Spot Price | P&L Without Stop-Loss | Adjusted P&L (With Stop-Loss) |
---|---|---|
₹21840 (Price based on Target) | +₹40 (Target Profit) | +₹40 |
₹21800 or ₹22200 (Breakeven) | 0 | 0 |
₹21700 or ₹22300 (Stop-Loss Triggered) | Unlimited | -₹100 (Capped) |
If the price stays between ₹21800 and ₹22200, the trade remains profitable. Beyond ₹22300 or below ₹21700, the stop-loss limits losses to -₹100 (₹200 - ₹300).
2. High-Risk Straddle Strategy
The diagram below illustrates the profit and loss structure for the high-risk variation of the short straddle strategy:
Figure 3: Profit and Loss Diagram : High Risk Version
Key Parameters:
- Target Profit (target_percentage): 35% of the total premium received
- Stop-Loss (stop_loss_multiplier): 1.5 x the total premium received
- Instrument: NVIDIA stock
- ATM Options Expiry Type: Nearest Monthly Expiry
Calculations:
-
Total Premium Received: $10 + $10 = $20
-
Target Profit: $20 x 0.35 = $7 So, we exit when the profit reaches +$7
-
Stop-Loss (1.5 * Total Premium Received):
-
Max Loss Capped at: -$10.
P&L Outcomes:
Underlying Spot Price | P&L Without Stop-Loss | Adjusted P&L (With Stop-Loss) |
---|---|---|
$87 (Price based on Target) | +$7 (Target Profit) | +$7 |
$80 or $120 (Breakeven) | 0 | 0 |
$70 or $130 (Stop-Loss Triggered) | Unlimited | -$10 (Capped) |
This version has a higher potential profit but a higher risk. If the price moves sharply, we lose more before the stop-loss activates.
Customise the Strategy with Your Own Parameters!
Every trader has a unique risk appetite and profit targets. If you find the parameters that suit your trading style but would like to customise further with your own data, feel free to reach out to us!
We can help you fine-tune this approach based on your specific needs.
👉 For testing on the Phoenix platform of AlgoBulls, head over to our site now!
👉 A Jupyter notebook for this strategy is coming soon. Meanwhile, check out All Available Notebooks for Testing Custom Strategies!
Final Thoughts
Beyond automated exits, traders can enhance risk control using trailing stops and real-time P&L tracking, adapting dynamically to market movements.
Key Risk Mitigation Techniques:
✔ Incorporated in the Strategy:
- Position adjustment: Modifies trade positions based on target price thresholds to maintain controlled risk exposure.
- Premium-based stop mechanism: Trades are exited when the premium exceeds the predefined stop-loss level.
✔ Additional Steps for Enhanced Risk Management:
- Trailing stops: Adjust dynamically to lock in gains as the trade moves favorably. Similar to how our strategy uses a stop-loss mechanism, this is a conditional approach to managing risk but is not a stop-loss order.
- P&L Tracking: Monitors profit and loss at a higher frequency than the candlestick interval, allowing timely adjustments. For example, it can update every 30 seconds for 1-minute candles. This is a built-in feature when developing out-of-the-box strategies on the AlgoBulls platform.
The Short Straddle Strategy offers structured returns in range-bound markets with predefined risk controls. Traders can choose Low-Risk (controlled losses) or High-Risk (greater exposure, higher profit potential) based on risk tolerance.